Java
Create a log
Be sure to check out the Get Started section for information on how to register for an account and create your first log. While creating your log choose “Java Log4J” or "Java Logback" option in “Language and framework” select field.
Below you will find integration instructions for Log4j and Logback.
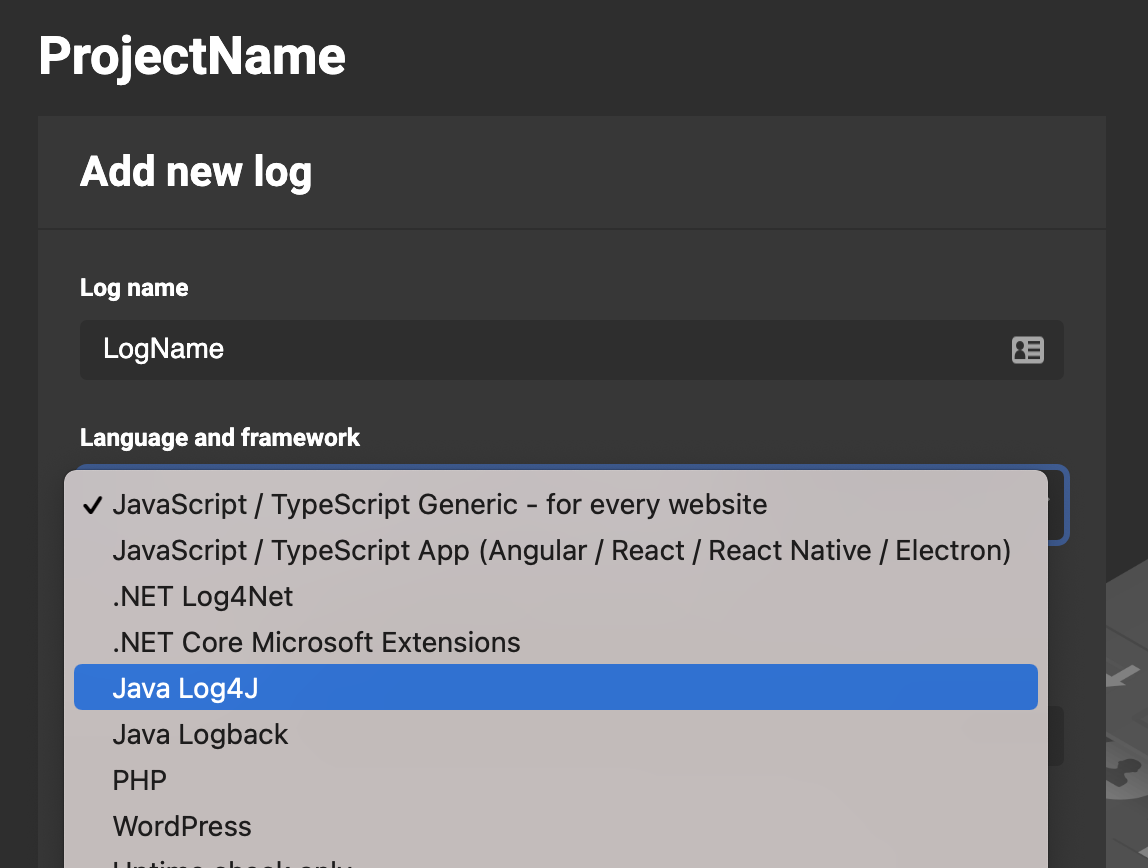
Log4j integration
See source code of example working application
To integrate Muscula with Log4j add dependency to POM file using this code:
<dependency>
<groupId>com.muscula</groupId>
<artifactId>muscula-log4j</artifactId>
<version>1.0.2</version>
</dependency>
Then configure Log4j in file src/main/resources/log4j2.xml
.
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="INFO">
<Appenders>
<MusculaAppender name="MusculaAppender" logId="LOG_ID"/>
</Appenders>
<Loggers>
<Root level="error">
<AppenderRef ref="MusculaAppender"/>
</Root>
</Loggers>
</Configuration>
Remember to replace LOG_ID with your current LOG ID. If you place code directly from Muscula Application LOG ID will be filled. Click here to see instructions on how to find your LOG ID in Mucula App.
To see a working example of log4j integration of Muscula check out our demo project at https://gitlab.com/muscula-public/muscula-java-log4j-demo.
Logback integration
See source code of example working application
To integrate Muscula with Logback add dependency to POM file using this code:
<dependency>
<groupId>com.muscula</groupId>
<artifactId>muscula-logback</artifactId>
<version>1.0.2</version>
</dependency>
Then configure logback in file src/main/resources/logback.xml
.
<configuration>
<appender name="muscula" class="com.muscula.logback.appender.MusculaAppender">
<logId>LOG_ID</logId>
</appender>
<root level="info">
<appender-ref ref="muscula"/>
</root>
</configuration>
Remember to replace LOG_ID with your current LOG ID. If you place code directly from Muscula Application LOG ID will be filled. Click here to see instructions on how to find your LOG ID in Mucula App.
You can use it like any other logger. Here is an example:
public class Main {
private static final Logger logger = LoggerFactory.getLogger(Main.class);
public static void main(String[] args) {
for(int i=0; i<10; i++)
logger.error("test", new RuntimeException("logback error test"));
}
}
To see a working example of logback integration check out our demo project at https://gitlab.com/muscula-public/muscula-java-logback-demo.
If you can’t find what you're looking for, contact us and we will update the documentation. Thanks, and enjoy Muscula!
Structural logging
You can pass any data alongside your log message. On example below we are sending additional data alongside error.
private static final Logger logger = LoggerFactory.getLogger(Main.class);
// sending info log with custom object
logger.info(
"Info log",
new MyObject("Application", "ConsoleManager")
);
// sending trace log with multiple custom named parameters
logger.trace(
"Trace log", new LogParam("ConsoleManager",
new MyObject("ProductIntegrationRequestId", 2049382)),
new LogParam("data", new ExampleData("AsyncTask", "ExportTaskExecutor"))
);
// seding error log with exception and custom parameters using MusculaThrowableProxy class
logger.error(
"Error log",
new MusculaThrowableProxy(new IllegalArgumentException("Invalid Request ID"),
new Request("ProductIntegrationRequestId", 2049382))
);
After this you will see in error details all data sent:
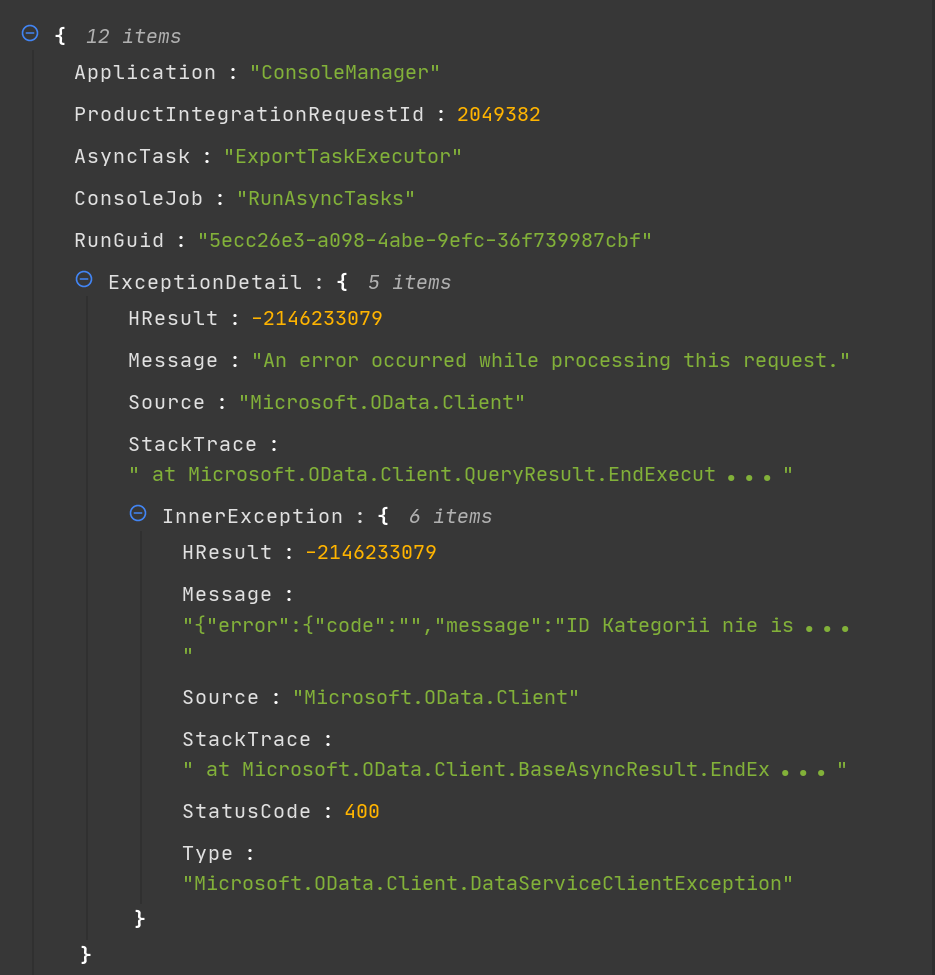