C#
Create a log
Be sure to check out the Get Started section for information on how to register for an account and create your first log. While creating your log choose “.NET Log4Net” or ".NET Core Microsoft Extensions" option in “Language and framework” select field.
Below you will find integration instructions for Microsoft Extensions Logging and Log4Net.
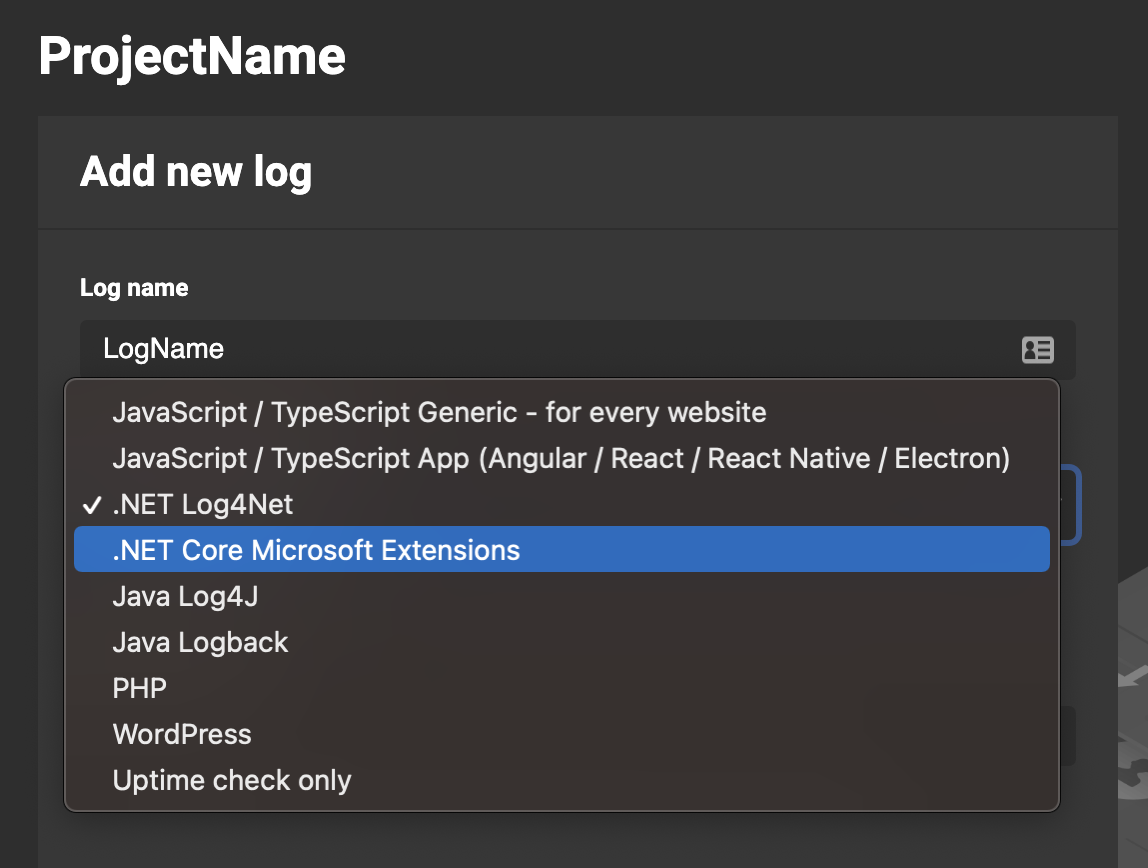
Installation for Microsoft Extensions Logging
See source code of example working application
Install this NuGet package: Muscula.Csharp.Extensions.Logging.AspNetCore. Visit this link to see package installation commands for Package Manager, .NET CLI, PackageReference, Paket CLI, Script & Interactive and Cake.
After installation, initialize logger at Program.cs using the code below:
if (!builder.Environment.IsDevelopment())
{
builder.Logging.AddMuscula((settingsBuilder) =>
{
settingsBuilder.UseLogId("<LOG_ID>");
return settingsBuilder.Build();
});
}
For .NET <6 Initialize logger at Startup.cs using the code below
public void ConfigureServices(IServiceCollection services) //this method should be there already
{
services.AddLogging(builder => builder.AddMuscula(
settingsBuilder => settingsBuilder.UseLogId(<LOG_ID>)
.UseStructuralLogger()
.Build()));
}
Remember to replace LOG_ID with your current LOG ID. If you place code directly from Muscula Application LOG ID will be filled. Click here to see instructions on how to find your LOG ID in Mucula App.
Installation for Log4Net
See source code of example working application
Install this NuGet package: Muscula.Csharp.Extensions.Logging.Log4Net. Visit this link to see package installation commands for Package Menager, .NET CLI, PackageReference, Paket CLI, Script & Interactive and Cake.
After installation, provide log4net.config file.
Remember to replace "LOG_ID" with your current LOG ID. If you place code directly from Muscula Application LOG ID will be filled. Click here to see instructions on how to find your LOG ID in Mucula App.
<?xml version="1.0" encoding="utf-8" ?>
<log4net>
<appender name="MusculaAppender"
type="Muscula.Csharp.Extensions.Logging.Log4Net.MusculaAppender, Muscula.Csharp.Extensions.Logging.Log4Net" >
<layout type="log4net.Layout.PatternLayout">
<conversionPattern value="%date [%thread] %-5level %logger [%ndc] - %message%newline" />
</layout>
<MusculaSettings>
<LogId value="LOG_ID" />
</MusculaSettings>
</appender>
<root>
<level value="ALL" />
<appender-ref ref="MusculaAppender" />
</root>
</log4net>
Then initialize logger at Startup.cs using this code:
public void ConfigureServices(IServiceCollection services) //this method should be there already
{
services.AddLogging(b => b.AddLog4Net("log4net.config"));
}
If you can’t find what you're looking for, contact us and we will update the documentation. Thanks, and enjoy Muscula!
Structural logging
You can pass any data alongside your log message. On example below we are sending additional data alongside error.
[ApiController]
[Route("[controller]")]
public class HomeController : ControllerBase
{
private readonly ILogger<HomeController> _logger;
private readonly IMusculaStructuralLogger _musculaLogger;
public HomeController(ILogger<HomeController> logger, IMusculaStructuralLogger musculaLogger)
{
_logger = logger;
_musculaLogger = musculaLogger;
}
[HttpGet("/demodata")]
public IActionResult LogDemoData()
{
var data = new DemoData();
data.Application = "ConsoleManager";
data.ProductIntegrationRequestId = 2049382;
_musculaLogger.LogStructural(Severity.Trace, "User entered demo data section", structuralData: data);
return Ok("OK");
}
After this you will see in error details all data sent:
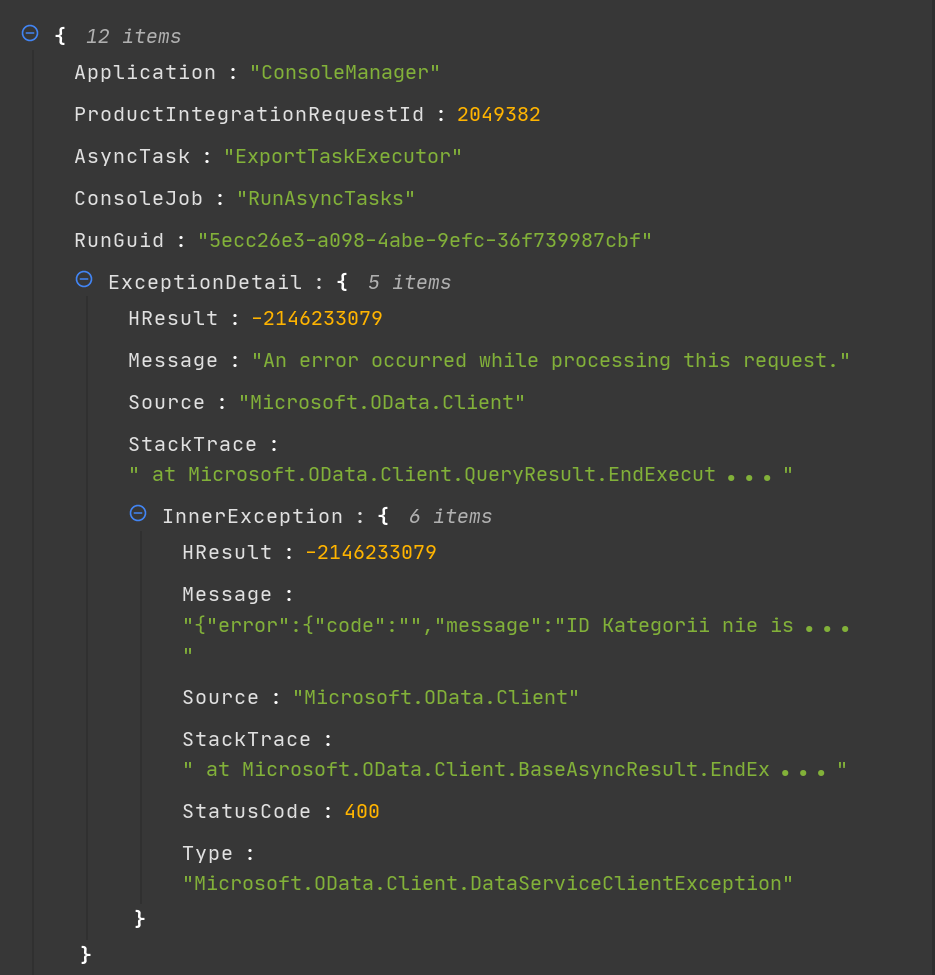